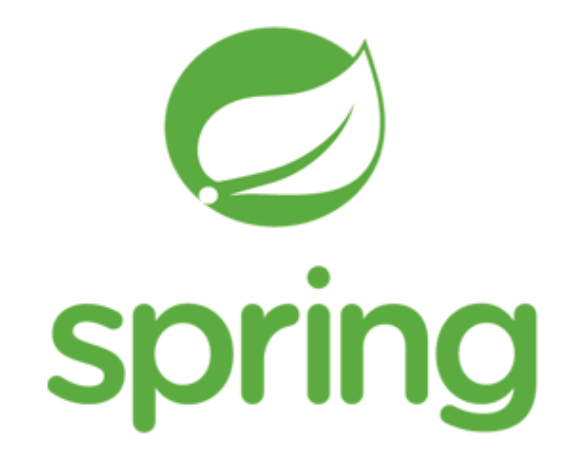
👉🏻 message.yml 이란?
messages.yml 파일은 Spring Framework, 특히 Spring Boot 애플리케이션에서 국제화(i18n)를 지원하기 위해 사용됩니다.
국제화는 애플리케이션이 여러 언어와 지역에서 사용될 수 있도록 지원하는 기능입니다. 이 파일을 통해 애플리케이션의 메시지(텍스트)를 여러 언어로 쉽게 관리하고 제공할 수 있습니다.
👉🏻 주요 이유 및 사용 목적
1. 국제화 및 지역화 지원:
• 애플리케이션을 여러 언어로 제공하여 글로벌 사용자에게 적합한 경험을 제공합니다.
• 지역별로 다른 언어와 문화에 맞춘 메시지를 쉽게 관리할 수 있습니다.
2. 중앙집중식 메시지 관리:
• 애플리케이션에서 사용되는 모든 메시지를 한 곳에서 관리할 수 있습니다.
• 코드와 메시지를 분리하여 유지보수성과 확장성을 높입니다.
3. 동적 언어 변경 지원:
• 사용자 요청에 따라 언어를 동적으로 변경할 수 있습니다.
• 예를 들어, 사용자가 언어를 선택하면 해당 언어로 메시지를 표시할 수 있습니다.
👉🏻 사용 예시
1. messages.yml 파일 생성
src/main/resources 디렉토리에 messages.yml 파일을 생성합니다. 예를 들어, 기본 메시지 파일과 다른 언어 메시지 파일을 생성할 수 있습니다.
• messages.yml (기본)
• messages_en.yml (영어)
2. messages.yml 파일 내용 예시
messages.yml (기본 메시지 파일)
greeting: 안녕하세요, 세계!
farewell: 안녕히 가세요!
messages_en.yml (영어 메시지 파일)
greeting: Hello, World!
farewell: Goodbye!
3. Spring Boot 설정
Spring Boot가 messages.yml 파일을 인식하도록 application.properties 또는 application.yml 파일에 설정을 추가합니다.
application.properties
spring.messages.basename=messages
spring.messages.file-extension=yml
또는
application.yml
spring:
messages:
basename: messages
file-extension: yml
4. 메시지 사용
컨트롤러나 서비스에서 메시지를 사용하려면 MessageSource를 주입받아 사용합니다.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.MessageSource;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.Locale;
@RestController
@RequestMapping("/api")
public class MessageController {
@Autowired
private MessageSource messageSource;
@GetMapping("/greet")
public String greet(@RequestParam(name = "lang", required = false) String lang) {
Locale locale = lang != null ? new Locale(lang) : Locale.getDefault();
return messageSource.getMessage("greeting", null, locale);
}
}
5. 테스트
이제 /api/greet 엔드포인트를 호출하여 메시지를 테스트할 수 있습니다. 예를 들어:
• 기본 메시지: http://localhost:8080/api/greet
• 영어 메시지: http://localhost:8080/api/greet?lang=en
이렇게 하면 요청한 언어에 맞는 메시지를 반환하게 됩니다.
'Framework > Spring' 카테고리의 다른 글
[Spring] RESTful API 컨트롤러의 @GetMapping 에서 데이터를 받는 방법 (0) | 2024.07.03 |
---|---|
[Spring] Spring Boot 기본, 자주 사용하는 어노테이션 (애너테이션) (0) | 2024.07.02 |
[Spring Boot] application.yml이란? application.yml 설정 방법 (0) | 2024.06.29 |
[Spring Boot] application.properties와 application.yml 차이 (0) | 2024.06.29 |
[Spring] @Controller와 @RestController의 차이 (0) | 2024.06.29 |
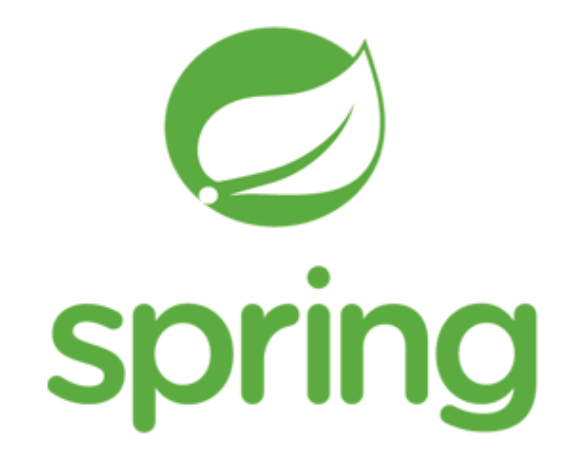
👉🏻 message.yml 이란?
messages.yml 파일은 Spring Framework, 특히 Spring Boot 애플리케이션에서 국제화(i18n)를 지원하기 위해 사용됩니다.
국제화는 애플리케이션이 여러 언어와 지역에서 사용될 수 있도록 지원하는 기능입니다. 이 파일을 통해 애플리케이션의 메시지(텍스트)를 여러 언어로 쉽게 관리하고 제공할 수 있습니다.
👉🏻 주요 이유 및 사용 목적
1. 국제화 및 지역화 지원:
• 애플리케이션을 여러 언어로 제공하여 글로벌 사용자에게 적합한 경험을 제공합니다.
• 지역별로 다른 언어와 문화에 맞춘 메시지를 쉽게 관리할 수 있습니다.
2. 중앙집중식 메시지 관리:
• 애플리케이션에서 사용되는 모든 메시지를 한 곳에서 관리할 수 있습니다.
• 코드와 메시지를 분리하여 유지보수성과 확장성을 높입니다.
3. 동적 언어 변경 지원:
• 사용자 요청에 따라 언어를 동적으로 변경할 수 있습니다.
• 예를 들어, 사용자가 언어를 선택하면 해당 언어로 메시지를 표시할 수 있습니다.
👉🏻 사용 예시
1. messages.yml 파일 생성
src/main/resources 디렉토리에 messages.yml 파일을 생성합니다. 예를 들어, 기본 메시지 파일과 다른 언어 메시지 파일을 생성할 수 있습니다.
• messages.yml (기본)
• messages_en.yml (영어)
2. messages.yml 파일 내용 예시
messages.yml (기본 메시지 파일)
greeting: 안녕하세요, 세계!
farewell: 안녕히 가세요!
messages_en.yml (영어 메시지 파일)
greeting: Hello, World!
farewell: Goodbye!
3. Spring Boot 설정
Spring Boot가 messages.yml 파일을 인식하도록 application.properties 또는 application.yml 파일에 설정을 추가합니다.
application.properties
spring.messages.basename=messages
spring.messages.file-extension=yml
또는
application.yml
spring:
messages:
basename: messages
file-extension: yml
4. 메시지 사용
컨트롤러나 서비스에서 메시지를 사용하려면 MessageSource를 주입받아 사용합니다.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.MessageSource;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.Locale;
@RestController
@RequestMapping("/api")
public class MessageController {
@Autowired
private MessageSource messageSource;
@GetMapping("/greet")
public String greet(@RequestParam(name = "lang", required = false) String lang) {
Locale locale = lang != null ? new Locale(lang) : Locale.getDefault();
return messageSource.getMessage("greeting", null, locale);
}
}
5. 테스트
이제 /api/greet 엔드포인트를 호출하여 메시지를 테스트할 수 있습니다. 예를 들어:
• 기본 메시지: http://localhost:8080/api/greet
• 영어 메시지: http://localhost:8080/api/greet?lang=en
이렇게 하면 요청한 언어에 맞는 메시지를 반환하게 됩니다.
'Framework > Spring' 카테고리의 다른 글
[Spring] RESTful API 컨트롤러의 @GetMapping 에서 데이터를 받는 방법 (0) | 2024.07.03 |
---|---|
[Spring] Spring Boot 기본, 자주 사용하는 어노테이션 (애너테이션) (0) | 2024.07.02 |
[Spring Boot] application.yml이란? application.yml 설정 방법 (0) | 2024.06.29 |
[Spring Boot] application.properties와 application.yml 차이 (0) | 2024.06.29 |
[Spring] @Controller와 @RestController의 차이 (0) | 2024.06.29 |