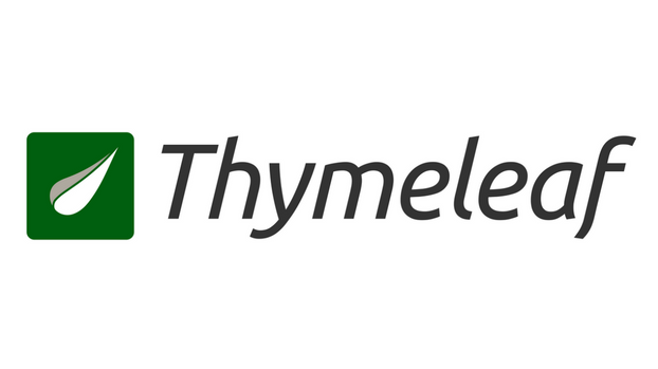
Thymeleaf(타임리프)란?
Java 기반의 템플릿 엔진으로, 웹 애플리케이션 서버 측에서
HTML, XML, JavaScript, CSS 등의 리소스를 동적으로 생성할 수 있도록 해줍니다.
주로 Spring Framework와 함께 사용되며, Spring MVC와 통합하여 뷰 레이어를 구성하는 데 자주 사용됩니다.
Thymeleaf의 다양한 기능과 장점
- HTML 친화적: Thymeleaf는 HTML 파일을 거의 그대로 유지하면서 동적 콘텐츠를 삽입할 수 있도록 설계되었습니다. 이는 브라우저에서 HTML 파일을 직접 열어도 올바르게 렌더링된다는 것을 의미합니다.
- 표현식 언어: Thymeleaf는 th:text, th:href, th:if 등의 속성을 통해 데이터 바인딩과 논리 처리를 지원합니다. 이를 통해 서버 측 데이터를 HTML에 쉽게 바인딩할 수 있습니다.
- Spring과의 통합: Thymeleaf는 Spring Framework와 자연스럽게 통합되어, Spring MVC의 모델 데이터를 뷰에 쉽게 전달할 수 있습니다.
- 다양한 템플릿 모드: Thymeleaf는 HTML5, XML, 텍스트, JavaScript, CSS 등의 다양한 템플릿 모드를 지원하여 다양한 종류의 템플릿을 처리할 수 있습니다.
- 확장성: Thymeleaf는 플러그인 아키텍처를 가지고 있어, 사용자 정의 다이얼렉트(dialects)를 추가하여 기능을 확장할 수 있습니다.
Thymeleaf를 사용하면 HTML 파일을 설계하는 동안 백엔드 데이터를 쉽게 통합하고, 뷰 로직을 서버 측에서 관리할 수 있어, 유지 보수성과 생산성이 향상됩니다. 이는 웹 애플리케이션 개발에서 특히 유용합니다.
Spring Boot Thymeleaf(타임리프) 설정 방법
1. 프로젝트 생성
Spring Initializr를 사용하여 Spring Boot 프로젝트를 생성합니다.
- Spring Initializr로 이동합니다.
- 프로젝트 메타데이터를 입력합니다 (예: Group, Artifact 등).
- Dependencies에서 Thymeleaf와 Spring Web을 선택합니다.
- Generate 버튼을 눌러 프로젝트를 다운로드합니다.
2. Maven 또는 Gradle 설정
생성된 프로젝트의 pom.xml 파일 또는 build.gradle 파일에 Thymeleaf 의존성을 추가합니다. Spring Initializr를 사용하여 프로젝트를 생성한 경우, 이 단계는 자동으로 설정됩니다. 하지만 수동으로 추가해야 하는 경우는 다음과 같습니다.
Maven (pom.xml)
<dependencies>
<!-- Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- Spring Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Gradle (build.gradle)
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
implementation 'org.springframework.boot:spring-boot-starter-web'
}
3. Thymeleaf 템플릿 파일 생성
src/main/resources/templates 디렉토리 아래에 Thymeleaf 템플릿 파일을 생성합니다. 예를 들어, index.html 파일을 생성할 수 있습니다.
src/main/resources/templates/index.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Thymeleaf Example</title>
</head>
<body>
<h1>Hello, <span th:text="${name}">World</span>!</h1>
</body>
</html>
4. Spring Boot 컨트롤러 생성
Spring Boot 애플리케이션의 컨트롤러를 생성하여 템플릿에 데이터를 전달합니다.
src/main/java/com/example/demo/HelloController.java
package com.example.demo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("/")
public String index(Model model) {
model.addAttribute("name", "Spring Boot");
return "index"; // This refers to the index.html in src/main/resources/templates
}
}
5. 애플리케이션 실행
Spring Boot 애플리케이션을 실행합니다. IDE를 사용하거나 명령어를 사용하여 애플리케이션을 실행할 수 있습니다.
명령어 사용 (Maven)
./mvnw spring-boot:run
명령어 사용 (Gradle)
./gradlew bootRun
브라우저에서 http://localhost:8080에 접속하면 index.html 템플릿이 렌더링된 페이지를 확인할 수 있습니다.
추가 설정 (선택 사항)
Spring Boot의 application.properties 파일에서 Thymeleaf와 관련된 추가 설정을 할 수 있습니다. 예를 들어, 캐싱을 비활성화하거나, 템플릿 파일의 인코딩 설정 등을 할 수 있습니다.
src/main/resources/application.properties
spring.thymeleaf.cache=false
spring.thymeleaf.prefix=classpath:/templates/
spring.thymeleaf.suffix=.html
spring.thymeleaf.mode=HTML5
이제 Spring Boot에서 Thymeleaf를 성공적으로 설정하고 사용할 수 있습니다.
'Programming > Thymeleaf' 카테고리의 다른 글
[Thymeleaf] 타임리프 레이아웃 분리 방법 (0) | 2024.06.16 |
---|---|
[Thymeleaf] 타임리프 HTML5 기본 구조 (0) | 2024.06.16 |